12 juin 2024
AI and UI #1 - Smart Filters with Vercel AI SDK and Next.js

4 minutes de lecture
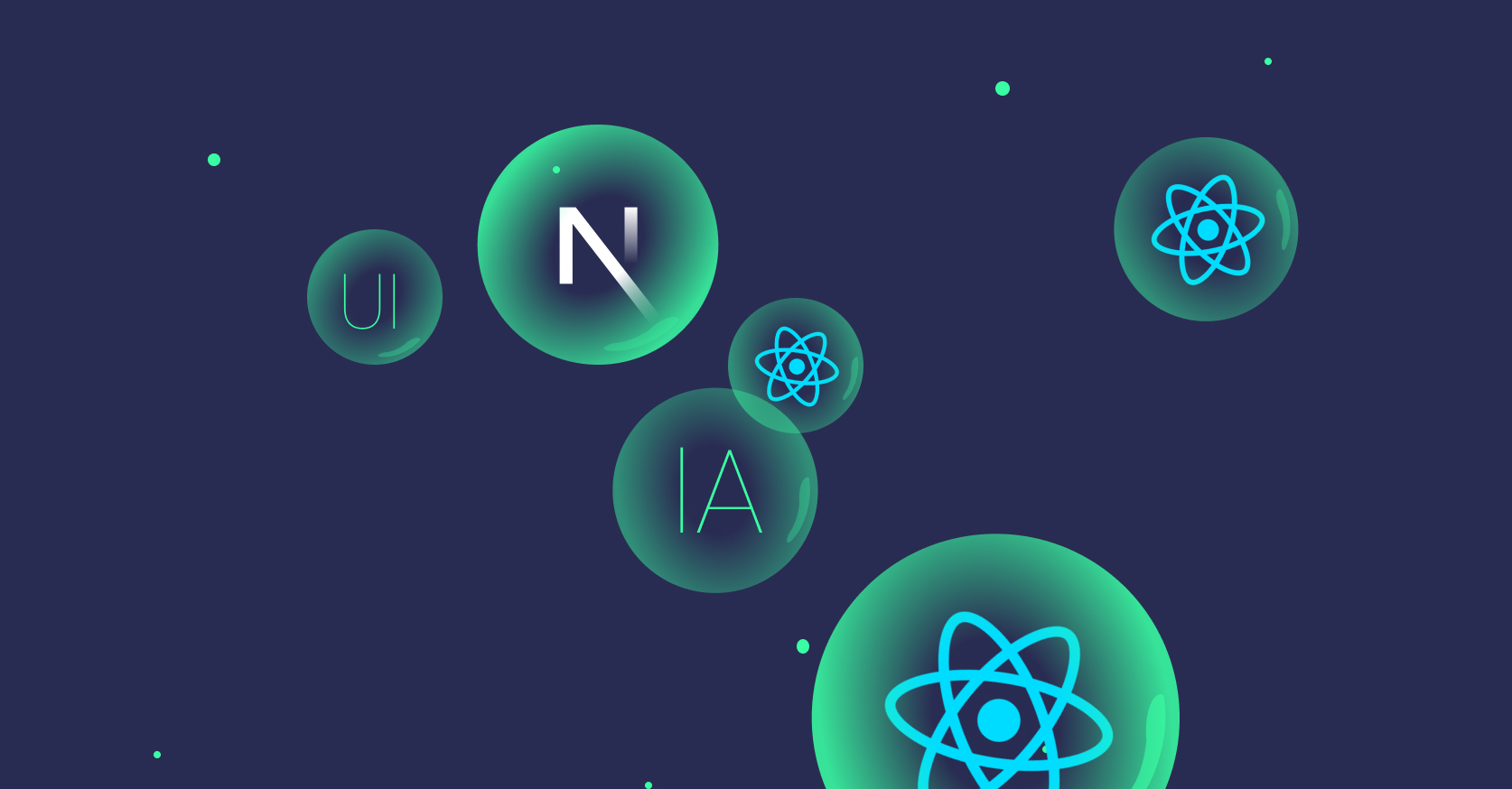
In this first article of a series dedicated to AI and UI, I propose to explore various ways to integrate these AI models into your React applications to enhance the user experience.
Similar to micro-interactions in the user experience, the concept of AI-boosted micro-interactions could be interesting to explore.
Smart Filters
Let's see how to integrate a smart filtering system into a Next.js application using the Vercel AI SDK. The idea is to allow the user to filter data using a simple description.
This feature, seen in products like Linear or Dub.co (see blog post), will be implemented in an isolated example that we will detail technically.
To start, here is a demo of what we will achieve:
The Filtering Interface
Here is our basic React interface:
A simple graph (using the Tremor library) displaying visits by day. Each visitor is associated with:
- a country
- a browser
- an operating system (OS)
We will introduce a search bar allowing to filter the data based on the user's description:
Zod Schema for Filters
We will use the Zod library (a TypeScript schema validation library) to define the schema of our filters.
Good news: the Vercel AI SDK will allow us to generate structured data from this Zod schema.
Here is our filtering system defined with Zod:
import z from 'zod'
const filtersSchema = z.object({
browser: z
.string()
.optional()
.transform((v) => v?.toLowerCase()),
country: z.enum(COUNTRY_CODES).optional(),
os: z
.string()
.optional()
.transform((v) => v?.toLowerCase()),
})
Country codes are retrieved from an array:
const COUNTRIES = {
FR: 'France',
JP: 'Japan',
// ...
}
const COUNTRY_CODES = Object.keys(COUNTRIES)
Now that we have defined our filtering system with Zod, let's see how to generate structured data from this schema using an LLM model.
Generating Structured Data
Most language models, such as GPT-4 (or Claude), can generate structured data in JSON format, making it easy to integrate into React or Next.js applications.
The Vercel AI SDK further simplifies this task by allowing the generation of structured data from a Zod schema using the generateObject function:
import { generateObject } from 'ai'
export async function generateFilters(prompt: string) {
const { object } = await generateObject({
model: openai('gpt-4o'),
schema: filtersSchema,
prompt: 'I want only japan users under Chrome',
})
return object
}
Let's see how GPT responds to the phrase “I want only Japan users under Chrome” while adhering to our schema:
I want only japan users under Chrome
{ "browser": "chrome", "country": "JP" }
Great! Let's test with another request:
show me mac french users on firefox
{ "browser": "firefox", "country": "FR", "os": "mac" }
The model indeed adds the missing properties and adheres to the Zod schema. We can now use this data to filter our graph.
Integration into the Application
We will create a server action to encapsulate our call to GPT-4 and generate the filters:
"use server";
import { COUNTRY_CODES } from "@/utils/utils";
import { openai } from "@ai-sdk/openai";
import { generateObject } from "ai";
export async function generateFilters(prompt: string) {
const { object } = await generateObject({
model: openai("gpt-4-turbo"),
schema: filtersSchema,
prompt,
});
return object;
}
We can now call this function in our Next.js page:
import { generateFilters } from '@/actions/generate-filters'
const Dashboard = () => {
const [filters, setFilters] = useState({})
const [prompt, setPrompt] = useState('')
const handleSubmit = async () => {
const filters = await generateFilters(prompt)
setFilters(filters)
}
return (
<form
onSubmit={(e) => {
e.preventDefault()
handleSubmit()
}}
>
<TextInput
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
placeholder="Your search..."
/>
<Button loading={isLoading}>Ask</Button>
</form>
)
}
All that’s left is to use the GPT response to filter your data and display it in your graph! Here is a sample code to filter the data:
const items = [
{ date: new Date('2024-01-01'), browser: 'chrome', country: 'FR', os: 'mac' },
{ date: new Date('2024-01-02'), browser: 'firefox', country: 'JP', os: 'linux' },
// ...
]
function filterItems(items, filters) {
if (filters?.length === 0) {
return items
}
return items.filter((item) => Object.keys(filters).every((key) => item[key] === filters[key]))
}
Here’s another look at the demo of what we achieved:
Key Takeaways
- Language models like GPT-4 can generate structured data in JSON format
- The Vercel AI SDK allows generating structured data from a Zod schema
- It is possible to integrate this structured data into a React / Next.js application to enhance the user experience
By combining AI with modern web development technologies, you can create more intuitive and efficient interfaces, offering an enriched and personalized user experience.
Code, Documentation, and Links
You can find the complete example code on our GitHub repo.
Here are some additional links for further reading on the topic:
- https://sdk.vercel.ai/docs/ai-sdk-core/generating-structured-data
- https://sdk.vercel.ai/docs/ai-sdk-core/schemas-and-zod
- https://x.com/steventey/status/1797671608347476412
Want to integrate AI into your React applications? Feel free to contact us to discuss it!
👋