15 avril 2025
View Transition API and its Integration in NextJS

6 minutes reading
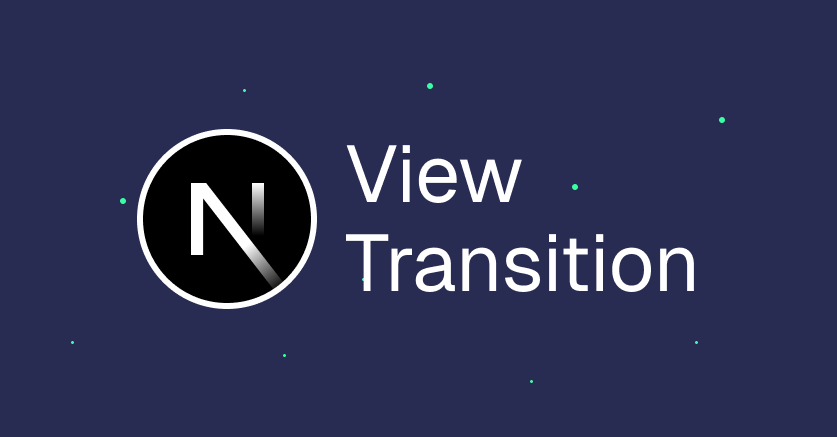
The View Transition API allows native animation of page state changes, without depending on third-party libraries. Recently, Next.js has experimentally integrated this feature.
Although this approach is still in the testing phase and minimally documented, it paves the way for simplifying animations that were once considered complex.
Before discussing its use in Next.js, I will briefly introduce the API in its native version for those who may not yet be familiar with it. If you are already familiar with the API, you can directly skip to this section.
What is the View Transition API?
The View Transition API is a native browser feature that allows animating DOM state or navigation changes without resorting to a third-party library like Framer Motion or GSAP. It significantly simplifies the integration of transitions/visual animations when updating a page's content.
This feature is not supported by all browsers. Currently, it's only supported by Chrome-based browsers and the Safari browser.
startViewTransition
The core of this API lies in the method startViewTransition
.
document.startViewTransition(() => updateTheDOMSomehow())
Calling this method, and passing it a callback function that updates the DOM, triggers a view transition cycle.
What does this mean? Essentially, when calling this method, the API captures the state of the page. Once the operation is complete (the page capture), your DOM update function is called and the API then again captures the state of the page, after your DOM mutation.
The API then builds a tree that looks like this:
::view-transition
└─ ::view-transition-group(root)
└─ ::view-transition-image-pair(root)
├─ ::view-transition-old(root)
└─ ::view-transition-new(root)
As the name suggests, ::view-transition-old
represents the capture of the old view and, as you may have guessed, ::view-transition-new
represents the current capture of the view.
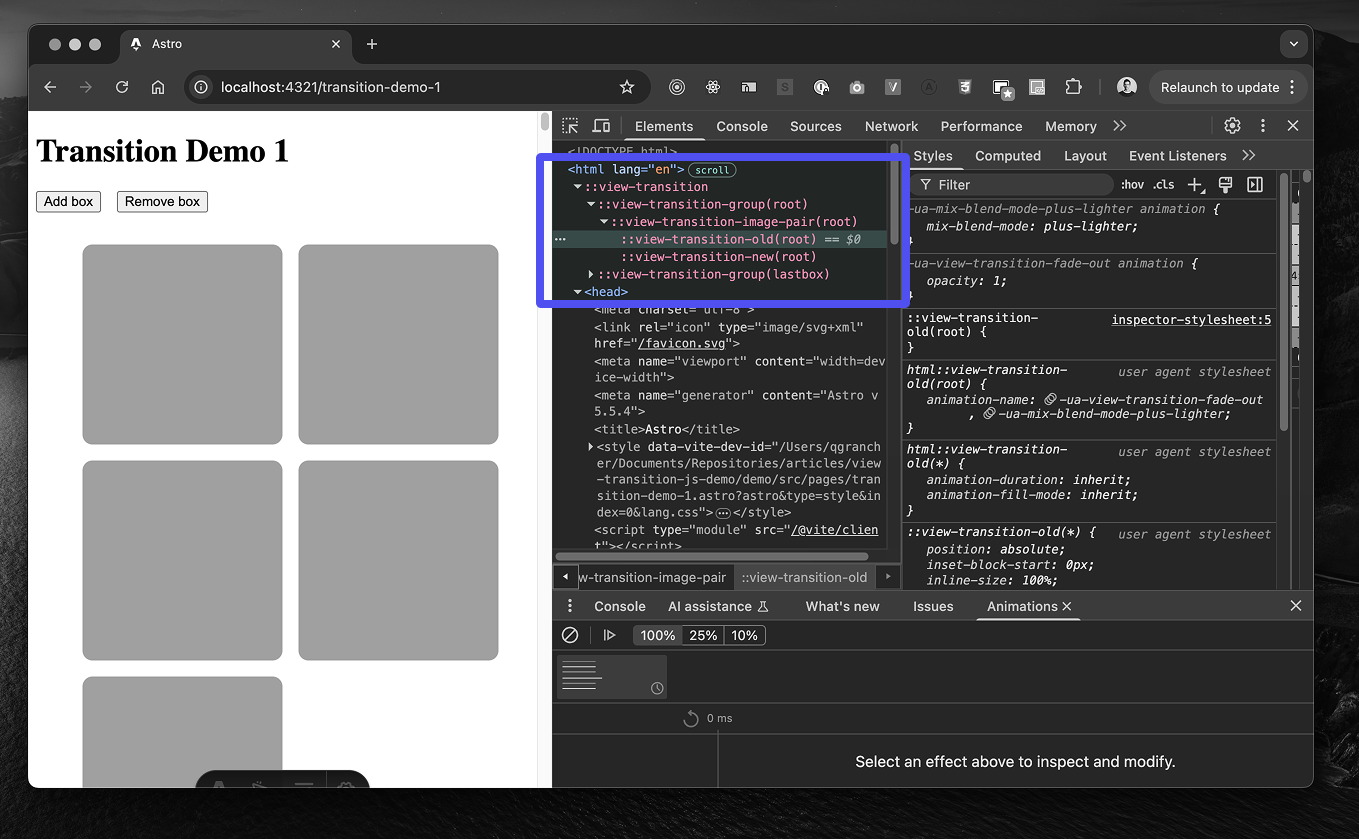
The old view is animated in fade out, while the new one appears in fade in (via the CSS property opacity
). This is the default behaviour, but it can of course be customised (this is the whole point).
Customising transitions
To customise view transitions, we will use the pseudo selectors ::view-transition....
in CSS.
So, if I want to make a slightly more complex transition (than a fade in/out), I can target my view transition and apply a CSS animation of my choice.
For example, here, I respectively apply my animations pop-in
and pop-out
that I would have defined earlier in my style sheet.
::view-transition-old(root) {
animation: pop-out 0.3s ease;
}
::view-transition-new(root) {
animation: pop-in 0.3s ease;
}
Target a specific element
In the previous example, we used a view transition on the whole page (root
), but it is possible to target a specific element.
To do this, we must first assign it a view transition name (view-transition-name
).
.box {
view-transition-name: box;
}
We can then target the view transition associated with this element with the corresponding pseudo elements:
::view-transition-old(box) {
animation: skew-out 0.3s ease;
}
::view-transition-new(box) {
animation: skew-in 0.3s ease;
}
You must assign a unique view-transition-name
to each element for the capture to work.
If you want to animate several elements in the same way, you can use the view-transition-class: myClass
property. In this case, you will need to prefix your class name with a period to select it with the pseudo-element (e.g., view-transition-old(.myClass)
or view-transition-new(.myClass)
).
Two Types of Transitions
View transitions fall into two categories.
Transitions on the same document and those on multi-documents (page change transition).
Both categories rely on the same principles, with the difference that for a multi-documents transition, there is no need to call the startViewTransition
method to start the transition. The navigation between the documents is what triggers the transition.
We are now generally up to date on the state of the View Transition API (as of the writing of this article).
If you wish to delve deeper into the API, I recommend the MDN documentation (although a little light), the W3C specification, and the many articles from Chrome For Developers on the subject.
Activating the Magic of Transitions in Next.js
Recently, a feature was introduced to facilitate the integration of the View Transition API in Next.js projects. But beware, we are stepping into very little documented territory here.
This feature, introduced in a release a few months ago, is still marked as experimental
. At the time of writing, the official documentation is quite minimal on the topic (here is the link if you want to take a look) and resources remain scarce.
It was precisely this lack of documentation that led me to dig a little bit deeper.
Digging around, I stumbled upon a demo shared by Delba Oliveira, a developer at Vercel. This made me want to delve deeper into the subject and experiment directly with this new API in a small test project on my end. Here's what I gleaned from it.
Spoiler: it's already promising.
To use this feature in NextJS, you need to enable the experimental flag viewTransition
. And use a version ≥ v15.2.0
.
module.exports = {
// ...
experimental: {
viewTransition: true,
},
}
The Component unstable_viewTransition
Currently, React exposes an unstable_viewTransition
component (yes, the name sets the tone). It takes several properties:
name
: Equivalent to theview-transition-name
in CSS.className
: To assign aview-transition-class
to the element.exit
/enter
: To add a class (CSS) for the exit or entrance animation of the element. (On mounting or unmounting the component)- And other properties that I've yet to explore but are typed and documented in the types of React.
In practical terms, here's what it might look like in the code:
import { unstable_viewTransition as ViewTransition } from 'react'
export default function MyComponent() {
return (
<ViewTransition name="box">
<div className="box">Hello</div>
</ViewTransition>
)
}
Even though the article here talks about integration in Next, it is crucial to note that this is fundamentally a feature of React introduced by this pull request.
This is why we import the component unstable_ViewTransition
from React.
A demonstration
And now... a little homegrown demo to show what it's like in action in a mini NextJS blog 👇
In this demo, we use the multi-document view transition, so it's the navigation that triggers the transitions.
I gave the same name
to the <ViewTransition>
component used in the /blog
page and the /blog/post/[slug]
page.
So, when navigating between the two pages, the transition is applied to animate the page change between these two elements.
Apart from a little blur effect on the images, this demo is purely accomplished with the React API, in default mode, as presented above, without any additional configuration. Therefore, it's relatively simple to implement.
And here is the source code used for this demo.
Limitations and Perspectives
The experimental integration of the View Transition API within Next.js opens up interesting prospects for developing smoother interfaces. In the future, we could imagine configurations of predefined transitions between pages, similar to NuxtJS.
However, as I have mentioned several times throughout this article, this is a feature that is not yet entirely production-ready. The API may change and will likely continue to evolve.
Bonus: Creative uses of the View Transition API
As a bonus, I've gathered some examples of sites that use the View Transition API creatively so you can see the possibilities it opens up.
- https://nmn.sh/
- https://cydstumpel.nl/
- https://x.com/delba_oliveira/status/1897701817431044124
- https://theme-toggle.rdsx.dev/
- https://framer-ground-svelte.vercel.app/layout/page-wipe